Overview
Error::Pure system is replacement for usage of Perl die
or Carp croak
.
Main features are:
Structured and defined output
Stack trace support inside
Basic usage
Simple Perl script, which have two subroutines and there is error inside. Error output via Error::Pure is to console and with ANSI colors. Output formatter is placed in "err_bt_pretty" in Error::Pure::Output::ANSIColor.
Environment set of output formatter
on bash
export ERROR_PURE_TYPE=ANSIColor::AllError
on tcsh
setenv ERROR_PURE_TYPE ANSIColor::AllError
Alternative way is add formatter to code
$Error::Pure::TYPE = 'ANSIColor::AllError';
Perl script
#!/usr/bin/env perl
use strict;
use warnings;
use Error::Pure qw(err);
sub first {
my $text = shift;
second($text);
return;
}
sub second {
my $text = shift;
err 'This is error',
'Context', $text,
;
}
first("Hello world");
Output to stderr
ERROR: This is error
Context: Hello world
main err /home/skim/data/gitprac/lang/perl/perl/MODULES/Error-Pure/ex19.pl 19
main second /home/skim/data/gitprac/lang/perl/perl/MODULES/Error-Pure/ex19.pl 11
main first /home/skim/data/gitprac/lang/perl/perl/MODULES/Error-Pure/ex19.pl 24
Output is in ANSI colors, you could look to image
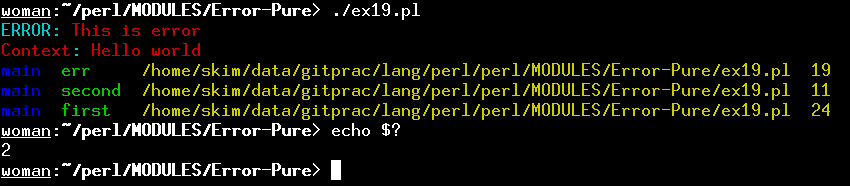
Capture error
The sama example as previous with capturing of error and print all information which we have. About fetching of information look to Error::Pure::Utils.
Perl script
#!/usr/bin/env perl
use strict;
use warnings;
use Data::Printer;
use English;
use Error::Pure qw(err);
use Error::Pure::Utils qw(err_get);
sub first {
my $text = shift;
second($text);
return;
}
sub second {
my $text = shift;
err 'This is error',
'Context', $text,
;
}
eval {
first("Hello world");
};
if ($EVAL_ERROR) {
print 'EVAL_ERROR: '.$EVAL_ERROR."\n";
my @errors = err_get();
p @errors;
}
Output
EVAL_ERROR: This is error
[
[0] {
msg [
[0] "This is error",
[1] "Context",
[2] "Hello world"
],
stack [
[0] {
args "('This is error', 'Context', 'Hello world')",
class "main",
line 22,
prog "/home/skim/err.pl",
sub "err"
},
[1] {
args "('Hello world')",
class "main",
line 14,
prog "/home/skim/err.pl",
sub "second"
},
[2] {
args "('Hello world')",
class "main",
line 28,
prog "/home/skim/err.pl",
sub "first"
},
[3] {
args "",
class "main",
line 27,
prog "/home/skim/err.pl",
sub "eval {...}"
}
]
}
]